How do I count the occurrences of each element in a vector
I came up to such questions when working on the Hardy-Ramanjan problem:
https://math.aalto.fi/opetus/Mattie/Blogi/Matlab/html/HardyRamanujan.html
After my way of counting using sort and diff presented above, I found this discussion with variuos interesting solutions to related problems. In this "publish-worksheet" I studied and ran through many of them for my own learnig and joy.
Contents
1. Logical indexing
x=[10 25 4 10 9 4 4] y = zeros(size(x)); for i = 1:length(x) y(i) = sum(x==x(i)); end y
x = 10 25 4 10 9 4 4 y = 2 1 3 2 1 3 3
2. hist
x=[10 25 4 10 9 4 4] [a,b]=hist(x,unique(x)) bar(b,a) %{ Solution 2 (using hist()) runs into trouble if unique(x) boils down to one number (a scalar). Then hist() takes it as the number of bins to use, not a bin center. Some if/else logic would catch this. Not sure if there is a one line answer. %} ux = unique(x); if length(ux) == 1, counts = length(x); else counts = hist(x,ux); end counts
x = 10 25 4 10 9 4 4 a = 3 1 2 1 b = 4 9 10 25 counts = 3 1 2 1
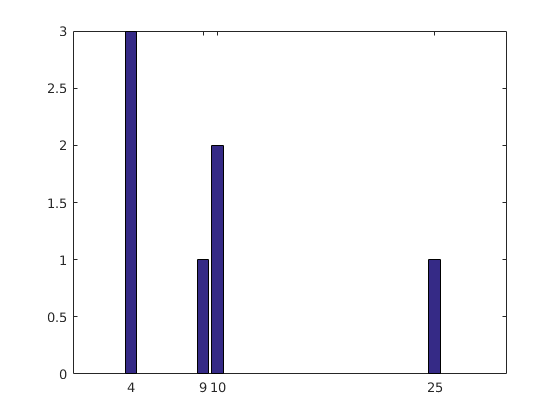
3. histc help
[N,BIN]=histc(x,unique(x)) % % [N,BIN] = histc(X,EDGES,...) also returns an index matrix BIN. If X is a % vector, N(K) = SUM(BIN==K) [N(BIN);x]
N = 3 1 2 1 BIN = 3 4 1 3 2 1 1 ans = 2 1 3 2 1 3 3 10 25 4 10 9 4 4
10 appears 2 times, 25 appears once, 4 appesra 3 times, ...
4. Solution with histc
[a,b] = histc(x,unique(x)); y = a(b)
y = 2 1 3 2 1 3 3
5. arrayfun, nnz
y = arrayfun(@(t)nnz(x==t), x)
%
y = 2 1 3 2 1 3 3
6. Variant: Count the number of occurrences of each integer 1,2,...
%{ I'm working with a small variant of the original problem, where I want it to count the number of occurrences of each whole number (till 13). So if my input is x = [1,1,1,2,4,5,5] I need an output y = [3,1,0,1,2] How do I do this? %} x = [1,1,1,2,4,5,5] y = accumarray(x(:),1)
x = 1 1 1 2 4 5 5 y = 3 1 0 1 2
6 another sol.
v=[1,1,1,2,4,5,5] numbers=unique(v) %list of elements count=hist(v,numbers) %provides a count of each element's occurrence % this will give counts. and if you want to have a nice graphical % representation then try this bar(accumarray(v', 1)) shg %{ When using hist() pay attention to Dan's comment above pointing out a flaw in the approach. This flaw is not shared by Andrei's histc approach above. %}
v = 1 1 1 2 4 5 5 numbers = 1 2 4 5 count = 3 1 1 2
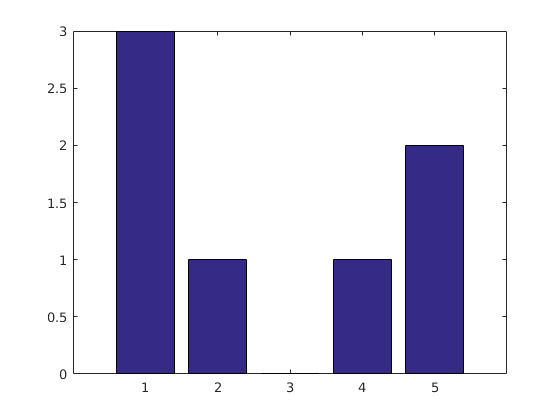